Add Search Functionality in ListView in Android
In this example , Today we are learning that how to add search functionality in ListView.
Implement Search Functionality in ListView will filters the list data with a matching string, So it will be easy for user to search any data very quickly from large data ListView.
Now We are discussing about how to implement search functionality in ListView
First of all, Create a new project from menu.For listview , we are using default xml file activity_main.xml and for Single list item create a new xml file named list_item.xml.
File Name : activity_main.xml
File Name : list_item.xml
Now, Open Your MainActivity.java file and copy the following code and paste in your file.
File Name : MainActivity.java
At the end , add the following property in your AndroidManifest.xml file
Output :
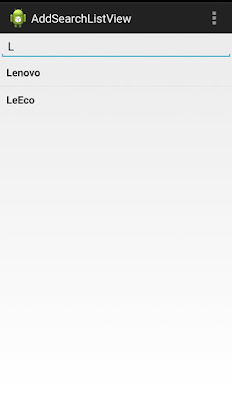
Implement Search Functionality in ListView will filters the list data with a matching string, So it will be easy for user to search any data very quickly from large data ListView.
Now We are discussing about how to implement search functionality in ListView
First of all, Create a new project from menu.For listview , we are using default xml file activity_main.xml and for Single list item create a new xml file named list_item.xml.
File Name : activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <EditText android:id="@+id/search" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="Search product" /> <ListView android:id="@+id/list_view" android:layout_width="fill_parent" android:layout_height="wrap_content" /> </LinearLayout> |
File Name : list_item.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <!-- List Item Of Product --> <TextView android:id="@+id/product_name" android:layout_width="fill_parent" android:layout_height="wrap_content" android:padding="10dip" android:textSize="16dip" android:textStyle="bold"/> </LinearLayout> |
Now, Open Your MainActivity.java file and copy the following code and paste in your file.
File Name : MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 | package com.example.addsearchlistview; import java.util.ArrayList; import java.util.HashMap; import android.app.Activity; import android.os.Bundle; import android.text.Editable; import android.text.TextWatcher; import android.view.Menu; import android.widget.ArrayAdapter; import android.widget.EditText; import android.widget.ListView; public class MainActivity extends Activity { ListView lv; ArrayAdapter<String> adapter; EditText search; ArrayList<HashMap<String, String>> productList; //ArrayList for Listview @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //Item of ListView String products[] = {"Lenovo", "Moto", "HTC", "iPhone", "Blackberry", "Samsung", "OnePlus", "LeEco", "Honor", "Micromax", "Xolo"}; lv = (ListView) findViewById(R.id.list_view); search = (EditText) findViewById(R.id.search); //Add Item in ListView adapter = new ArrayAdapter<String>(this, R.layout.list_item, R.id.product_name, products); lv.setAdapter(adapter); // To Enabling Search Functionality search.addTextChangedListener(new TextWatcher() { @Override public void onTextChanged(CharSequence cs, int arg1, int arg2, int arg3) { // When user changed the Text MainActivity.this.adapter.getFilter().filter(cs); } @Override public void beforeTextChanged(CharSequence arg0, int arg1, int arg2, int arg3) { // TODO Auto-generated method stub } @Override public void afterTextChanged(Editable arg0) { // TODO Auto-generated method stub } }); } } |
At the end , add the following property in your AndroidManifest.xml file
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.addsearchlistview" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="17" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.example.addsearchlistview.MainActivity" android:label="@string/app_name" android:windowSoftInputMode="stateHidden" > // To hide keyboard on loading activity <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> |
Output :
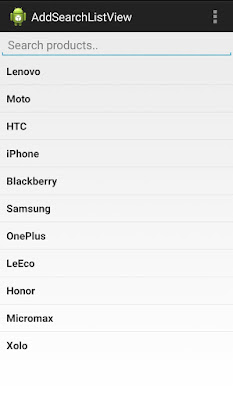
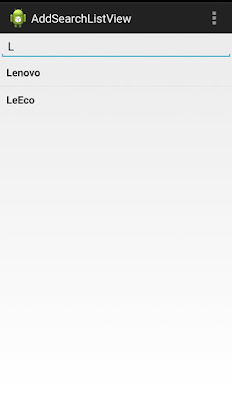
Add Search Functionality in ListView in Android, Android Tutorial, ListView, Search data from Listview in Android, Searching in ListView
Really Helpful article Sir. Thank you :)
ReplyDelete